Do you want to build a simple calculator using Python? Whether you are a beginner or an experienced programmer, building a calculator is a great way to practice your coding skills. In this blog post, we will show you the process of building a basic calculator using Python. After completing this guide, you will have a fully functional calculator that can perform basic mathematical operations such as addition, subtraction, multiplication, and division.
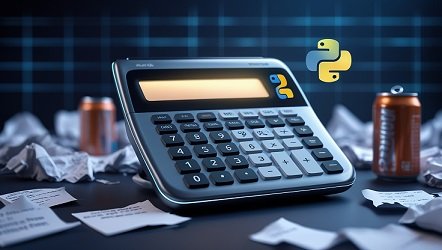
Why Build a Calculator in Python?
Python is one of the most popular programming languages, known for its simplicity and readability. It is an excellent choice for beginners and is widely used in various fields, including web development, data science, and automation. Building a calculator is a perfect project to gain first-hand experience with Python’s syntax and logic.
Prerequisites
Before starting the code, make sure you have the following:
- Python installed: Make sure you have Python installed on your computer. You can download it from the official Python website.
- Text editor or IDE: Use a text editor like VS Code, Sublime Text, or an IDE like PyCharm to write your code.
Step 1: Define the Basic Operations
First, let’s define the basic arithmetic operations that our calculator will support:
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
We’ll create functions for each of these operations.
python
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
return "Error! Division by zero."
return x / y
Step 2: Create the Calculator Interface
Next, we’ll create a simple text-based interface that allows the user to choose an operation and input two numbers.
python
def calculator():
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
choice = input("Enter choice (1/2/3/4): ")
if choice in ['1', '2', '3', '4']:
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print(f"{num1} + {num2} = {add(num1, num2)}")
elif choice == '2':
print(f"{num1} - {num2} = {subtract(num1, num2)}")
elif choice == '3':
print(f"{num1} * {num2} = {multiply(num1, num2)}")
elif choice == '4':
print(f"{num1} / {num2} = {divide(num1, num2)}")
else:
print("Invalid input")
# Run the calculator
calculator()
Step 3: Run Your Calculator
Save your Python script with a .py
extension, for example, simple_calculator.py
. To run the script, open your terminal or command prompt and navigate to the directory where your script is saved. Then, type:
python simple_calculator.py
You will see the calculator interface, and you can start calculating!
Step 4: Improve your calculator (optional)
Once you have your basic calculator working, you can enhance it by adding more features, such as:
- Handling more operations: Add support for exponentiation, square roots, etc.
- Error handling: Improve error messages and handle invalid inputs gracefully.
- GUI: Create a graphical user interface (GUI) using libraries like Tkinter or PyQt.
Conclusion
Building a simple calculator in Python is a fantastic way to practice your programming skills and understand the basics of Python. By following this guide, you’ve learned how to define functions, create a user interface, and perform basic arithmetic operations. As you continue your Python journey, you can expand on this project by adding more features or exploring other Python libraries.
Happy coding! If you found this guide helpful, feel free to share it with others who are learning Python. And don’t forget to check out our other Python tutorials for more exciting projects!